Quick Guide: In this javascript simulator, you will see a snippet of html element, followed by the code for that html element. There will be a textfield below that, with a 'RUN' button next to it. You can edit the text inside of the textfield, and it will run your input as javascript code and show you the result below. If the javascript code you entered has a visual effect, that will also be seen.
DOM Objects
elements:
You can use javascript to get the html elements inside of the webpage. document will give you all the whole html code of the webpage. document.body will give you the html code inside of and including the <body> tag of your webpage. You can get a more specific section of your html code by looking up the id of that element using the getElementById() function. Given that we have the particular html code below, try to figure out the javascript code for getting the <p> tag with text "Javascript is fun!".
Javascript is fun!
<div id="text-div"> <p id="div-content">Javascript is fun!</p> </div>
Examples of code for selecting HTML elements: You can try these in the above textfield!
document document.body //returns the HTML element with the id of ‘text-div’ document.getElementById('text-div') document.getElementById('div-content')
Properties of DOM Elements:
You can do more than just looking up html elements with javascript. You can also look up specific properties that pertain to an HTML element. You are already familiar with some properties of HTML elements, but you just not might think of them as such. In the section of HTML below, we have an image tag, with 3 attributes: id, width and src. The attributes of an HTML element, that get written inside of the HTML tag, are part of properties of the HTML element. Therefore, you can look up the values of these properties of an HTML tag, as well as set them as some other value of your choice.
My Title
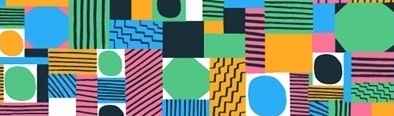
<h1 id="title">My Title!</h1> <img id="pattern" width="100%" src="img/img1.jpg">
Examples of code for looking up properties of an <img> element:
document.getElementById('pattern').id document.getElementById('pattern').width document.getElementById('pattern').height //if the HTML element is an image, returns the source (file path) if the image document.getElementById('pattern').src
Examples of code for looking up properties of general HTML elements:
document.getElementById('title').id //returns the width of the HTML element //the width includes the width of the content, padding-left and padding-right document.getElementById('title').clientWidth //returns the height of the HTML element //the height includes the height of the content, padding-top and padding-bottom document.getElementById('title').clientHeight //returns the content inside of the HTML element document.getElementById(title').innerHTML
Examples of code for setting properties of an <img> element:
Note that in my img folder, there are 5 images called: img1.jpg, img2.jpg, img3.jpg, img4.jpg and img5.jpg.
document.getElementById('pattern').id = "new-id" document.getElementById('pattern').width = 200 document.getElementById('pattern').height = 500 //if the DOM element is an image, sets the source (file path) if the image document.getElementById('pattern').src = "img/img2.jpg"
Examples of code for setting properties of general HTML elements:
document.getElementById('title').id = "new-id" //sets the content inside of the HTML element document.getElementById('title').innerHTML = "New text for my h1 tag!"
element.style:
You can also look up the CSS styles applied to a particular HTML element as well as set them to a value of your choice. To refer to the css related to a particular HTML element, simply add .style after. If you want to look up / set a value of a particular CSS property, add another . (dot) and follow it by that CSS property. If the CSS property you want to look up / set has a - (dash) in its name, change the way it is written using camel casing. Camel casing is a way of writing compound / multiple words that usually replaces spaces, but in this cas dashs, by capitalizing the word that would usually come after the space / dash. For example, background-color can be rewritten as backgroundColor. Use the HTML code below to look up and set CSS properties in the textfield.
Javascript is fun!
HTML:
<div id="text-box"> <p id="inner-text">Javascript is fun!</p> </div>
CSS:
#text-box{ background-color: black; color: white; padding: 24px; } #inner-text{ font-size: 24px; text-align: center; }
Examples of code for looking up CSS properties of an HTML element:
document.getElementById('text-box').style.backgroundColor document.getElementById('text-box').style.color document.getElementById('text-box').style.padding document.getElementById('inner-text').style.fontSize document.getElementById('inner-text').style.textAlign
Examples of code for setting CSS properties of an HTML element:
document.getElementById('text-box').style.backgroundColor = "red" document.getElementById('text-box').style.color = "#cecece" document.getElementById('inner-text').style.fontSize = "48px"
BOM Objects
Properties of BOM Objects:
Examples of code for looking up properties of window object:
//returns the width of the browser window window.innerWidth //returns the height of the browser window window.innerHeight //returns the x position of the scroll window.scrollX //returns the y position of the scroll window.scrollY
Methods of BOM Objects:
Examples of code for using methods of window object:
//window.scrollTo(xpos, ypos) //scrolls the window to xpos from the left and ypos from the top //xpos and ypos are in pixels window.scrollTo(0, 100) //window.scrollBy(xnum, ynum) //scrolls by xnum amount towards the right and ynum amount towards the bottom every time this function is triggered //xnum and ynum are in pixels window.scrollBy(10, 50)
Examples of code for creating different styles of popup boxes:
//displays the alert message alert("This is an alert message!") //opens a confirm popup window with ‘OK’ and ‘Cancel’ buttons confirm("Please press OK to confirm!") //opens a prompt popup window with a place for users to enter in text prompt("What is your name?")
Example code for creating a popup window:
//window.open(url, name, specs); //name: _blank, _parent, _self, _top //specs: width (px), height (px), top (px), left (px) //to use specs, you must have a name argument window.open('http://ima.nyu.sh', '_blank', 'width=200, height=200, left=500, top=100')
Code for closing the current window:
window.close()
DOM Events
Mouse Events:
function clickEvent(){ document.getElementById('click-div').style.backgroundColor = "red"; } function dblclickEvent(){ document.getElementById('click-div').style.backgroundColor = "blue"; } function onmouseoverEvent(){ document.getElementById('click-div').style.border = "solid 5px green"; } function onmouseoutEvent(){ document.getElementById('click-div').style.border = "dotted 10px yellow"; }
Keyboard Events:
When you type, the keys you press will be shown here!
function onkeypressEvent(){ document.getElementById('keyInput').innerHTML = "You pressed: " + event.key; } function onkeydownEvent(){ document.getElementById('keyBox').style.backgroundColor = "pink"; } function onkeyupEvent(){ document.getElementById('keyBox').style.backgroundColor = "lightblue"; }